Selling ‘offline’ products with WooCommerce

One of the e-commerce websites I look after sells a lot of heavy-duty equipment that requires installation by a specialist. Because I have no price set on some of the products, I need to show a call to action instead. This is purely for the purpose of generating leads.
Standard WooCommerce behaviour for a product with no price is to hide the add to cart button. Because of this, I had to build a plugin to add in a call-to-action box.
The WooCommerce Empty Price HTML Hook
It’s fairly straightforward due to WooCommerce’s excellent API, which makes it possible to extend most aspects of the WooCommerce plugin. ( There are a few instances I’ve wanted to do something and ended up pulling my hair out. However, in this case, I was all set. )
The code makes use of the woocommerce_empty_price_html hook, and here is a basic example of how it can be implemented:
<?php function chrisjallen_price_on_application() { // Get access to the $woocommerce_loop global $woocommerce_loop; // If there is no loop, its not a product page, or the loop has no name, then bail. if ( is_null( $woocommerce_loop ) || ! is_product() || strlen( $woocommerce_loop['name'] ) ) { return; } // Get the partial and use it. chrisjallen_get_template_part( 'partials/product', 'empty-price-box' ); } // Add the above function to the woocommerce_empty_price_html filter. add_filter( 'woocommerce_empty_price_html', 'chrisjallen_price_on_application' );
So, to summarise the above snippet:
- Gain access to the WooCommerce products loop.
- Check we are in the loop, and on a product page.
- Include the HTML we want to display.
- Attach the code to the woocommerce_empty_price_html hook.
The above example is very basic and requires you to hard-code your HTML into a template partial. It worked fine for my use-case, but I thought it would help if I enhanced the functionality & packaged it into a plugin.
Woo Custom Empty Price
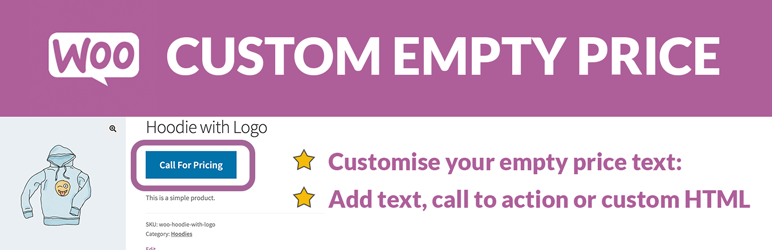
The Woo Custom Empty Price plugin allows you to add whatever you like, without any coding at all. ( Unless you choose the “Custom HTML” option. ) It gives you three simple content types and allows basic configuration out of the box. You also have an optional CSS class should you choose to roll your own styles.
Each content type is easily selectable via a dropdown, and selecting one will display the relevant options for that content type. I’ll explain the three types here:
Plain text
This is the simplest option of all. It’s a text box, with options for basic styling such as 3 preset font sizes, colour, font weight and italic options. You should find this fine for most cases when you don’t need a link. If you want to style the text yourself, use the default .cep-text class or choose your own.

Call to action
In the case where you require a button, choose ‘Call to action’ as your content type. You have options to set the appearance of the button. You’ll also find a destination URL setting for when the button is clicked. Again, should you require your own styling, you can add a custom CSS class or just style the default .cep-button class.

Custom HTML
The last content type is a WYSIWYG editor, so you can add pretty much whatever HTML you like. Just be aware that as standard, the WYSIWYG editor will not allow you to add script tags, so if you require Javascript functionality, then you’ll need to enqueue your own.

A note about variable products
I’ve designed this plugin primarily for ‘Simple’ products. It works with all types, but will only show if none of your product variations has a price set.
In this scenario, you will also see a built-in WooCommerce message saying “This product is currently out of stock and unavailable.“
You may not want to display this message, but the only decent way of hiding it is to directly edit your theme template. It’s the default WooCommerce behaviour and if it doesn’t suit you, then the easiest route is to just use a simple product.
Feedback wanted
This plugin is completely free to use, so I’d love it if you can offer feedback if you find it useful. I can use this feedback in future versions, as I’d like to add more features in the next release, such as support for modal pop-ups, custom CSS & the ability to enqueue your own dependency scripts.
If not feedback, then a review on WordPress.org helps massively. Enjoy!
Leave a Reply